Integrating AI-Powered Smart Filters in React Applications
The blog post explores how to integrate an AI-powered smart filtering system into a Next.js application using the Vercel AI SDK. The goal is to allow users to filter data using natural language descriptions.
The Filtering Interface
The application features a simple graph displaying visitor data, including country, browser, and operating system (OS). A search bar is introduced to allow users to filter the data based on their descriptions.
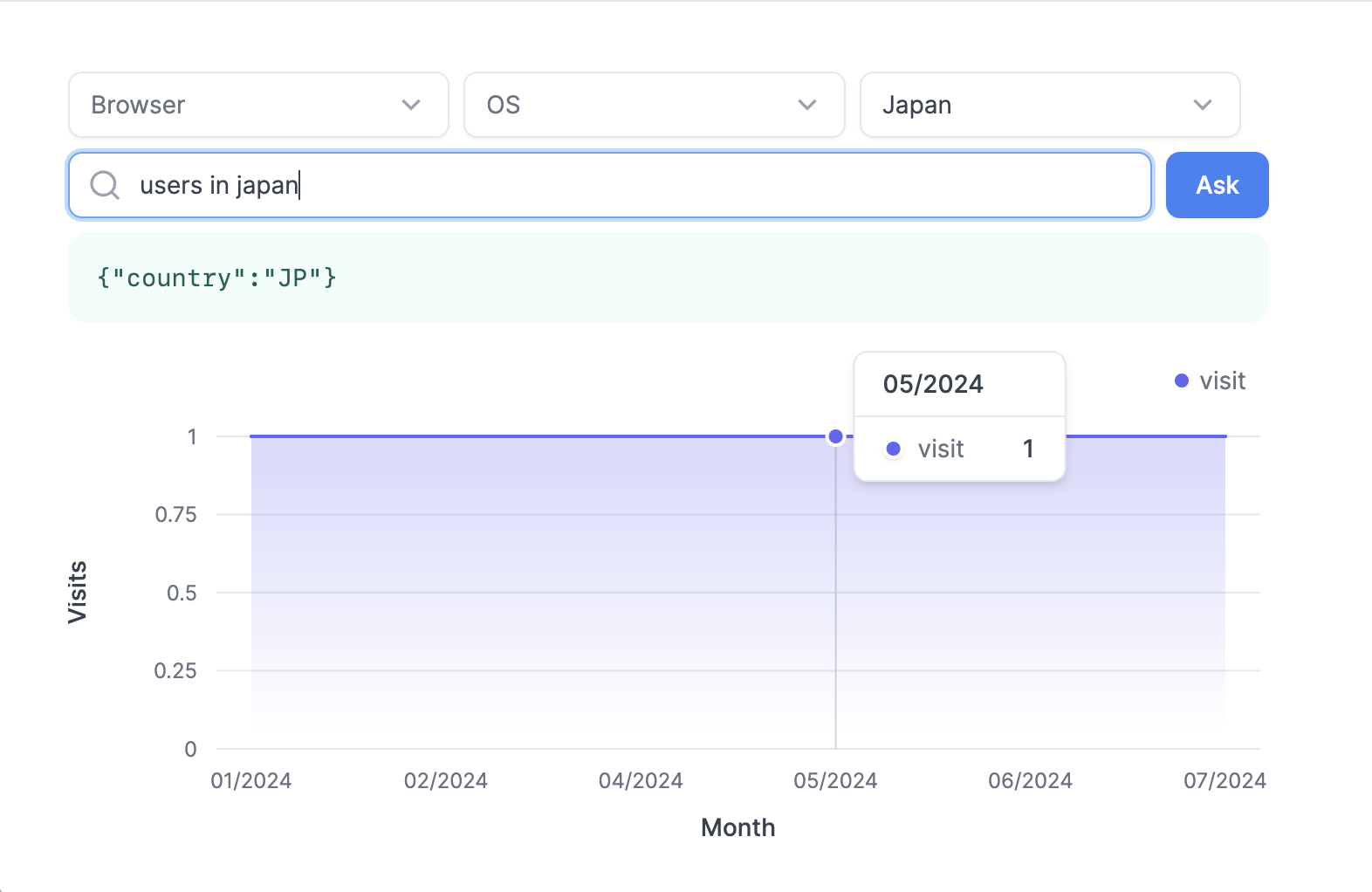
Zod Schema for Filters
We will use the Zod library to define the schema of the filters. This includes fields for browser, country, and OS.
const filtersSchema = z.object({
browser: z.string().optional().transform((v) => v?.toLowerCase()),
country: z.enum(COUNTRY_CODES).optional(),
os: z.string().optional().transform((v) => v?.toLowerCase()),
});
Generating Structured Data with Claude 3.5 Sonnet
The Vercel AI SDK is used to generate structured data from the Zod schema using a language model like Claude 3.5 Sonnet. This allows the application to interpret natural language prompts and return the corresponding filters.
export async function generateFilters(prompt: string) {
const { object } = await generateObject({
model: anthropic("claude-3-5-sonnet-20240620"),
schema: filtersSchema,
prompt,
system: `You are a filters generator.`+
`You generate filters based on the given JSON schema and user prompt.`
});
return object;
}
For example, the prompt "I want only Japan users under Chrome" generates the following structured data:
{ "browser": "chrome", "country": "JP" }
Integrating into the Application
We will create a server action to encapsulate our call to Claude 3.5 Sonnet and generate the filters:
"use server";
import { COUNTRY_CODES } from "@/utils/utils";
import { anthropic } from "@ai-sdk/anthropic";
import { generateObject } from "ai";
export async function generateFilters(prompt: string) {
const { object } = await generateObject({
model: anthropic("claude-3-5-sonnet-20240620"),
schema: filtersSchema,
prompt,
});
return object;
}
We can now call this function in our Next.js page:
import { generateFilters } from '@/actions/generate-filters'
const Dashboard = () => {
const [filters, setFilters] = useState({})
const [prompt, setPrompt] = useState('')
const handleSubmit = async () => {
const filters = await generateFilters(prompt)
setFilters(filters)
}
return (
<form
onSubmit={(e) => {
e.preventDefault()
handleSubmit()
}}
>
<TextInput
value={prompt}
onChange={(e) => setPrompt(e.target.value)}
placeholder="Your search..."
/>
<Button loading={isLoading}>Ask</Button>
</form>
)
}
All that’s left is to use the Claude response to filter your data and display it in your graph! Here is a sample code to filter the data:
const items = [
{ date: new Date('2024-01-01'), browser: 'chrome', country: 'FR', os: 'mac' },
{ date: new Date('2024-01-02'), browser: 'firefox', country: 'JP', os: 'linux' },
// ...
]
function filterItems(items, filters) {
if (filters?.length === 0) {
return items
}
return items.filter((item) => Object.keys(filters).every((key) => item[key] === filters[key]))
}
Key Takeaways
The blog post highlights the following key points:
- Language models like Claude 3.5 Sonnet can generate structured data in JSON format.
- The Vercel AI SDK allows generating structured data from a Zod schema.
- Integrating AI-powered features can enhance the user experience in React/Next.js applications.